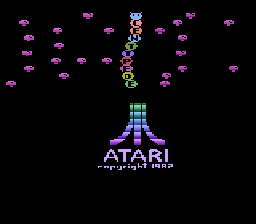
There are two interesting things about this game. The first is it uses a finite state machine, the second is the method by which the centipede moves.
Globals
Declares assorted globals. They are initialised in Event 4 (this is a one restart layout = one set of lives thing). There are some utility functions to update the lives, the score,, add a value to a score. Event 11 is used to randomise the colour on the little score thing that appears when you shoot some things (hence "On created").
Event 12-15 colours an object in the Colourable family by UID, replacing colour 5 (white in this scenario) with orange and adding a "white override" - this is because the poisoned mushrooms are white. The actual colour is derived from the level number. The colour works by adding a SetColor effect in WebGL (hence it requires WebGL to provide the colour). You could do this by having different frames/animations in the different colours but that's not so much fun as playing with WebGL.
Mushrooms
Most of the code here is concerned with initialisation - it creates equally spaced mushrooms at the top, this is to break up the centipede parts early. Duplicate mushrooms are created at event 5 (I think I forgot to put the While in there .... oops). Events 5 and 6 handle the shooting and destroying of mushrooms (which require 3 hits each), and 7 scores when you finally beat the mushroom.
Finally event 8 causes any new mushroom to be coloured appropriately as per above.
Player
Event 2 initialises the positions, Event locks it in a "player area" at the bottom of the screen, and Event 4 fires a missile if the Ctrl key is pressed. Easy enough so far .... well it isn't now :)
Centipede
The heart of it. Each centipede is a singly linked list effectively, with the "nextUIDInChain" instance variable creating a list. The head of the list, which is also the head of the centipede is marked as such (isLeader instance variable).
It moves as follows. Each segment moves under its own code to a new "target" coordinate, but this target coordinate is passed down the list. So the head tells segment 1 to go where it is, segment 1 tells segment 2 to go where it is and so on.
Event 2 detects being shot. This splits the linked list, which becomes two seperate ones - event 3 stores the UID of the head of the new list. Event 4 and 5 do the scoring, and create a new mushroom. Evemt 7, destroying the segment, finally breaks the list (the previous entry now points to a non existent UID). The new head is then selected and made up to be a leader (8 and 9).
Events 10-17 create a centipede of a given size. They are created with all the segments on top of each other (12 picks a spot). 13 and 14 create the body, keeping the uid of the previous part of the chain in "uid", and 15 is the same except it just does the head.
Event 18 onwards moves the centipede(s). It does it with the leaders first (in 18) so that the orders are set up in the correct order.
Event 19 detects if the leader has reach its target. If it has, it calls the "PassNewTargetDown" function which causes each segment in the list to pass its current position on to the next segment as its new target.
Event 20 causes the reversal on hitting a mushroom, or being poisoned, or a collision(21) or being on the edge of the screen (22,23). If none of these applies the new target is one position either space horizontally in the current direction (24). If there was a reversal it is to move down and once that has completed continuing moving horizontally (25).
Event 26 and 27 keep the centipede in the player area at the bottom, making it endlessly cycle in that area.
Event 28 fires for all, and moves the object towards its currently specified target. Event 29 shouldn't be there and doesn't do anything anyway.
Event 29 is a recursive function which passes the target down the linked list - all it does is copy the target from the parameters and then call it with the next segments stuff. I often wondered if recursion worked in C2. It does.
Event 32-37 creates Centipedes or centipede heads dependent on the level, at one of two speeds.
Spider Scorpion and Fleas
This is actually three seperate files really, one for each of the other centipede characters. They all start in a similar fashion with timers for random intervals (e.g. 2 and 3) and the creation starts at 4. The sine behaviour causes the spider's zig zag effect vertically and a simple chaser moves it horizontally, this is done in 5. Every so often the 'target' of the chaser is recalculated by 6.
Events 7 and 8 deal with shooting it - the score depends on how far apart they are, and it spawns a transient score item and a particle effect.
Finally 9 and 10 turn the spider tune off and on as a spider is created or destroyed (there is only one at once).
The Flea initial code is similar (12,13) except they only appear on level 2. The first thing it does is count all the mushrooms in the player area, and if there aren't enough, fires the flea. This drops down the screen using a bullet behaviour, and creates mushrooms on the way down. It stops at the bottom (18) and can be shot (19) with particles and transient score again.
The Scorpion again is very similar. Event 23 creates it (Level 3+) and it moves horizontally, event 24 allow s it to go the other way. Any mushrooms it collides with are poisoned (25) which causes the centipede to crash to the bottom of the screen. They can be destroyed in event 26, but this also unpoisons all mushrooms on the same line (event 27), which isn't strictly accurate as it should only unpoison the ones its just poisoned, but its near enough.
State
This handles the four states of the game. In "new level" it creates a new level (basically centipedes) in 5, recolours all the objects in 6.
The main play state starts at 8. Hitting a segment or animal switches it to "life lost", and destroying all the segments switches it to a new (next) level in 10 and 11. 12 starts the thud thud thud noise.
Life lost starts at 15, where the basic adjustments are made, and the segments are all destroyed. Each mushroom flashes briefly which is set up by a timer in 17 and implemented in 18. When all these are completed (19) it switches either to new level (the same level again) or game over depending on whether there are any lives left, or not.
Finally, 23 and 24 implement the game over state which fades "Game over" onto the screen and restarts the layout when space is pressed.
Title Sheet
Events 3-5 create the wobbling centipede with the letters in (I did not use pin for this because it did not centre properly). Events 6-9 create a vague approximation of the Atari logo with horizontal lines, and 10 and 11 create the mushrooms
Each tick event 13 moves the letters with the circles, and 14 uses time to animate the raster bar. Finally 15 starts the game.
No comments:
Post a Comment